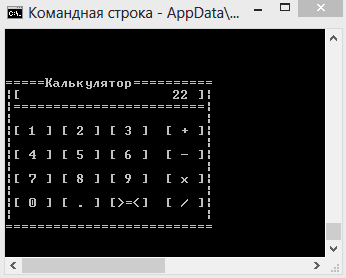
Управление: стрелки на клавиатуре и Enter, выход из программы — Esc
Исходник calc.py:
# -*- coding: utf-8 -*-
# kmsvsr.ru 2020-06-06
import msvcrt
from decimal import *
def makeDisplayString(s):
result = " " * (20 - len(s)) + s
return result
class MyCalculator:
def __init__(self):
self.last_result = Decimal('99999999999999999999.0')
self.result = Decimal('0.0')
self.display_default='''
=====Калькулятор==========
|[ 00000000000000000000 ]|
|========================|
| |
|[ 1 ] [ 2 ] [ 3 ] [ + ]|
| |
|[ 4 ] [ 5 ] [ 6 ] [ - ]|
| |
|[ 7 ] [ 8 ] [ 9 ] [ x ]|
| |
|[ 0 ] [ . ] [ = ] [ / ]|
| |
==========================
'''
self.display = self.display_default
self.keys=("123+", "456-", "789x", "0.=/")
self.ACTION_EMPTY = 0
self.ACTION_PLUS = 1
self.ACTION_MINUS = 2
self.ACTION_MULTIPLY = 3
self.ACTION_DIVIDE = 4
self.last_action = self.ACTION_EMPTY
self.visible = 1
self.display_digits = "0" # цифры, которые видно на калькуляторе, не больше 20
self.last_display_digits = ""
self.updateDigitsOnDisplay()
# положение курсора слева сверху
self.curx=0
self.cury=0
self.needNewDigit = 0 # 1 после кнопки действия
def updateKeysOnDisplay(self):
self.display = self.display.replace('[ %s ]' % self.keys[self.cury][self.curx], '[>%s<]' % self.keys[self.cury][self.curx])
def updateDigitsOnDisplay(self):
if len(self.display_digits) > 0:
self.display = self.display.replace('00000000000000000000', makeDisplayString(self.display_digits) )
else:
self.display = self.display.replace('00000000000000000000', makeDisplayString(self.last_display_digits) )
def resetDisplay(self):
self.display = self.display_default
def show(self):
self.visible = 1
self.redraw()
def redraw(self):
self.resetDisplay()
self.updateDigitsOnDisplay()
self.updateKeysOnDisplay()
if self.visible == 1:
print(self.display)
def calc(self):
try:
new_value = Decimal('%s' % self.display_digits)
except:
new_value = Decimal('99999999999999999999.0')
if self.last_action == self.ACTION_PLUS:
self.result = self.last_result + new_value
if self.last_action == self.ACTION_MINUS:
self.result = self.last_result - new_value
if self.last_action == self.ACTION_MULTIPLY:
self.result = self.last_result * new_value
if self.last_action == self.ACTION_DIVIDE:
self.result = self.last_result / new_value
if self.last_action == self.ACTION_EMPTY:
self.result = new_value
if self.last_action != self.ACTION_EMPTY:
self.display_digits = "%s" % self.result
self.last_result = self.result
self.needNewDigit = 1
self.last_display_digits = self.display_digits
'''
H
KPM
'''
calc = MyCalculator()
calc.show()
while True:
if calc.needNewDigit == 1:
calc.display_digits = ""
calc.needNewDigit = 0
lastkey = msvcrt.getch()
#print(lastkey)
if lastkey == b'\x1b': # Esc
break
if lastkey == b'K': # left
if calc.curx > 0:
calc.curx -= 1
calc.updateKeysOnDisplay()
if lastkey == b'P': # down
if calc.cury < 3:
calc.cury += 1
calc.updateKeysOnDisplay()
if lastkey == b'M': # right
if calc.curx < 3:
calc.curx += 1
calc.updateKeysOnDisplay()
if lastkey == b'H': # up
if calc.cury > 0:
calc.cury -= 1
calc.updateKeysOnDisplay()
if lastkey == b'\r':
#Разбираемся, какая кнопка нажата на калькуляторе
selected = calc.keys[calc.cury][calc.curx]
if calc.curx < 3 and calc.cury < 3: # цифры больше 0
newd = selected # новая нажатая цифра
if calc.display_digits == "0":
calc.display_digits = newd
elif len(calc.display_digits) < 20:
calc.display_digits += newd
if calc.curx == 0 and calc.cury == 3: # 0
if calc.display_digits != "0" and len(calc.display_digits) < 20:
calc.display_digits += selected
if calc.curx == 1 and calc.cury == 3: # . (точка)
if len(calc.display_digits) < 20 and '.' not in calc.display_digits:
calc.display_digits += selected
#.needNewDigit
#.last_action
if calc.curx == 3 and calc.cury == 0: # +
calc.calc()
calc.last_action = calc.ACTION_PLUS
if calc.curx == 3 and calc.cury == 1: # -
calc.calc()
calc.last_action = calc.ACTION_MINUS
if calc.curx == 3 and calc.cury == 2: # x
calc.calc()
calc.last_action = calc.ACTION_MULTIPLY
if calc.curx == 3 and calc.cury == 3: # /
calc.calc()
calc.last_action = calc.ACTION_DIVIDE
if calc.curx == 2 and calc.cury == 3: # =
calc.calc()
calc.last_action = calc.ACTION_EMPTY
print('\n'*25)
calc.redraw()
Скачать: Скачать