DECLARE @mesto varchar(30);
DECLARE c CURSOR FOR SELECT mesto from MyPlaces
OPEN c
FETCH NEXT FROM c INTO @mesto
WHILE @@FETCH_STATUS = 0
BEGIN
select 'Здесь ' + @mesto
FETCH NEXT FROM c INTO @mesto
END
CLOSE c
DEALLOCATE c
Архив метки: пример
Пример python time sleep
import time
time.sleep(5)
MSSQL результат — значения в строку через запятую
Пример запроса:
DECLARE @idName INT
SET @someColumn = 7
DECLARE @s VARCHAR(MAX)
SET @s = ''
SELECT @s = @s + CASE WHEN @s <> '' THEN ', ' ELSE '' END + CAST(myColumn AS VARCHAR)
FROM t1
WHERE someColumn = @someColumn
SELECT @s AS ResultList
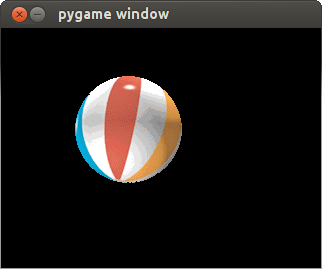
Python: заготовка игры с использованием pygame
Пример программы на Python с использованием библиотеки pygame. Создаётся объект из рисунка, прописаны события на нажатие и отпускание клавиш со стрелками. В цикл добавлена задержка sleep.
Код:
import sys, pygame pygame.init() size = width, height = 640, 480 speed = [0, 0] black = 0, 0, 0 screen = pygame.display.set_mode(size) ball = pygame.image.load("hero.gif") ballrect = ball.get_rect() while 1: for event in pygame.event.get(): if event.type == pygame.QUIT: sys.exit() if event.type == pygame.KEYDOWN: if event.key == pygame.K_UP: speed[1] -= 2 if event.key == pygame.K_DOWN: speed[1] += 2 if event.key == pygame.K_LEFT: speed[0] -= 2 if event.key == pygame.K_RIGHT: speed[0] += 2 if event.type == pygame.KEYUP: if event.key == pygame.K_UP: speed[1] = 0 if event.key == pygame.K_DOWN: speed[1] = 0 if event.key == pygame.K_LEFT: speed[0] = 0 if event.key == pygame.K_RIGHT: speed[0] = 0 ballrect = ballrect.move(speed) if ballrect.left < 0 or ballrect.right > width: #speed[0] = -speed[0] speed[0] = 0 if ballrect.top < 0 or ballrect.bottom > height: #speed[1] = -speed[1] speed[1] = 0 screen.fill(black) screen.blit(ball, ballrect) pygame.display.flip() pygame.time.delay(20)
md5deep
-r Enables recursive mode. All subdirectories are traversed. Please note that recursive mode cannot be used to examine all files
of a given file extension. For example, calling md5deep -r *.txt will examine all files in directories that end in .txt.
-e Displays a progress indicator and estimate of time remaining for each file being processed. Time estimates for files larger
than 4GB are not available on Windows. This mode may not be used with th -p mode.
-t Display a timestamp in GMT with each result. On Windows this timestamp will be the file’s creation time. On all other systems
it should be the file’s change time.
-z Enables file size mode. Prepends the hash with a ten digit representation of the size of each file processed. If the file
size is greater than 9999999999 bytes (about 9.3GB) the program displays 9999999999 for the size.
-o <bcpflsd>
Enables expert mode. Allows the user specify which (and only which) types of files are processed. Directory processing is
still controlled with the -r flag. The expert mode options allowed are:
f — Regular files
b — Block Devices
c — Character Devices
p — Named Pipes
l — Symbolic Links
s — Sockets
d — Solaris Doors
e — Windows PE executables
MS SQL Умножение Numeric без округлений
Более-менее рабочий пример, чтоб например 4.5 не превратилось в 5
DECLARE @tmp_cnt Numeric (18,10) DECLARE @tmp_uet Numeric (18,10) … @tmp_cnt = 1.0 @tmp_uet = 4.5 … CAST ( @tmp_cnt * @tmp_uet AS Numeric(18,10))
Всё работает из-за (18,10) (precision, scale)
Ещё подробности здесь:
Bash: как найти последний созданный в папке файл
Bash: как найти последний созданный в папке файл:
ls -t | head -1
Если нужно найти файл определённого типа, то можно вот так:
ls -t | grep jpg | head -1
C#: пример подключения к базе данных MSSQL
C#: пример подключения к базе данных MSSQL
/* * Created by SharpDevelop. * http://kmsvsr.ru * Date: 11.04.2015 * Time: 15:23 */ using System; using System.Data.SqlClient; namespace ExecSQL { class Program { public static void Main(string[] args) { Console.WriteLine("Let`s execute our query!"); using (SqlConnection con = new SqlConnection("user id=sa;password=Pa$$w0rd;server=192.168.3.4;database=MyBase;connection timeout=30;")) { con.Open(); using (SqlCommand command = new SqlCommand("select COLUMN1 from MYTABLE where COLUMN2 ='http://kmsvsr.ru'", con)) using (SqlDataReader reader = command.ExecuteReader()) { while (reader.Read()) { //Console.WriteLine("{0} {1} {2}", reader.GetInt32(0), reader.GetString(1), reader.GetString(2)); Console.WriteLine("{0}", reader.GetInt32(0)); } } } Console.Write("Press any key to exit . . . "); Console.ReadKey(true); } } }
Выполнение команд Powershell из CMD или .bat
Команды Powershell можно выполнять из коммандной строки CMD или скриптов .bat следующим образом:
I:\>powershell "get-psdrive | ft name" > test.txt
Linux: как заархивировать папку
zip -9 -r ./folder.zip ./folder
Здесь -9 — степень сжатия, а -r — рекурсия для содержимого папки